In trading platforms, you can request buy or sell orders on a financial asset.
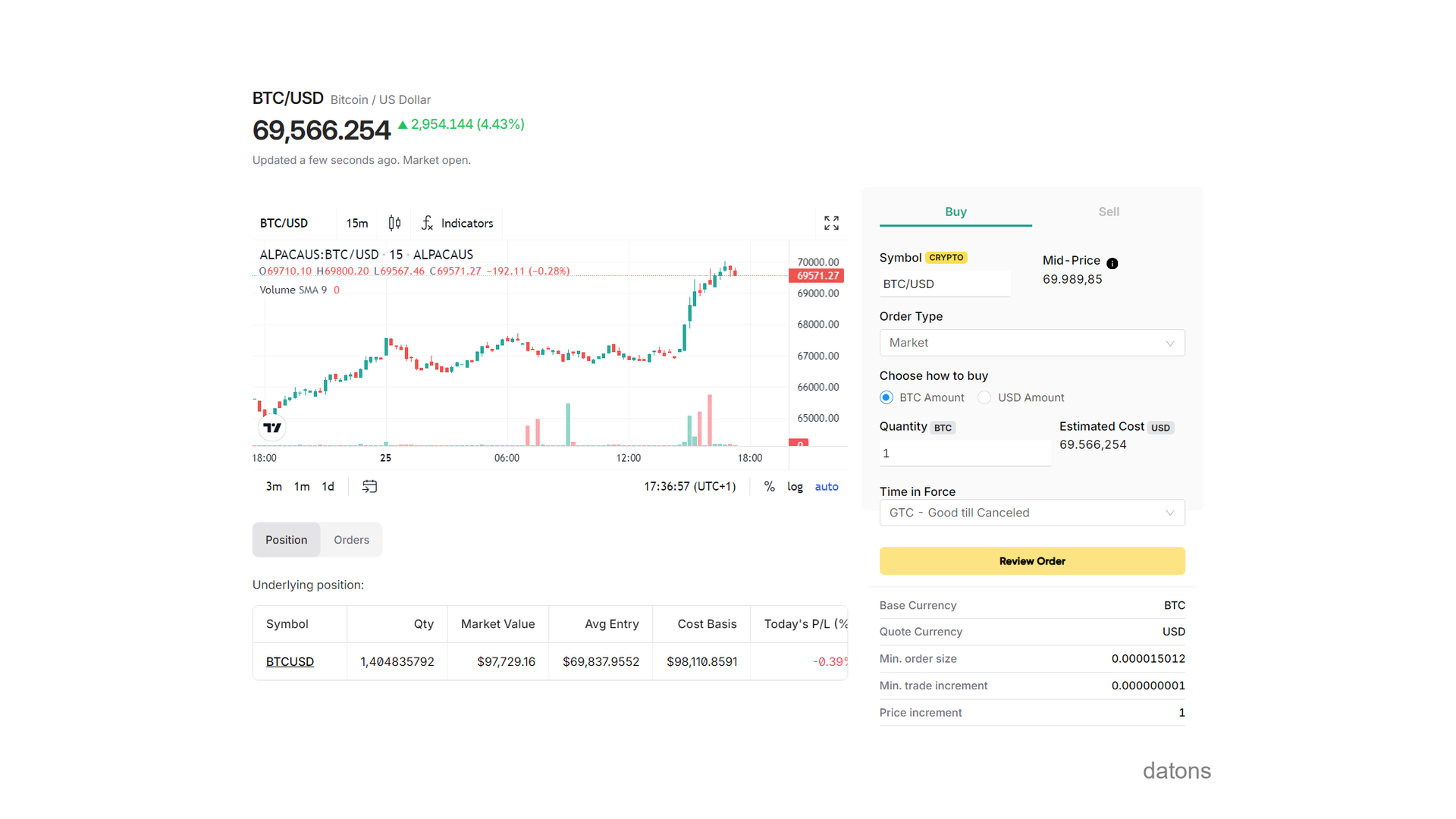
You can program a bot to execute your orders to automate your operations.
In this tutorial, you’ll learn to request orders programmatically with the Alpaca API using a demo account.
Disclaimer: This tutorial is for educational purposes to teach you to program with practical cases. It is not an investment recommendation.
Questions
- How do you authorize the code to execute trading operations with your Alpaca account?
- What general information do you have about your demo account?
- How do you create a buy order for a financial asset?
- What does the
GTC
parameter mean in a buy order? Why is it important? - How do you close an open position on a specific asset?
- How do you view updated information on your portfolio after performing operations?
Methodology
Create an Account on Alpaca
Register at Alpaca and generate an API Key to validate your programmatic access.
To avoid risks, do not register any payment method when creating your account.
API_KEY = 'YOUR_API_KEY'
API_SECRET = 'YOUR_API_SECRET'
Account Properties
Once you have installed the Alpaca library:
pip install alpaca-py
You create the trading client instance in demo mode,
paper=True
, to validate your connection to the API.
from alpaca.trading.client import TradingClient
client = TradingClient(API_KEY, API_SECRET, paper=True)
Request your account information to verify that everything is in order.
data = client.get_account()
You start with a balance of $100,000 in your demo account.
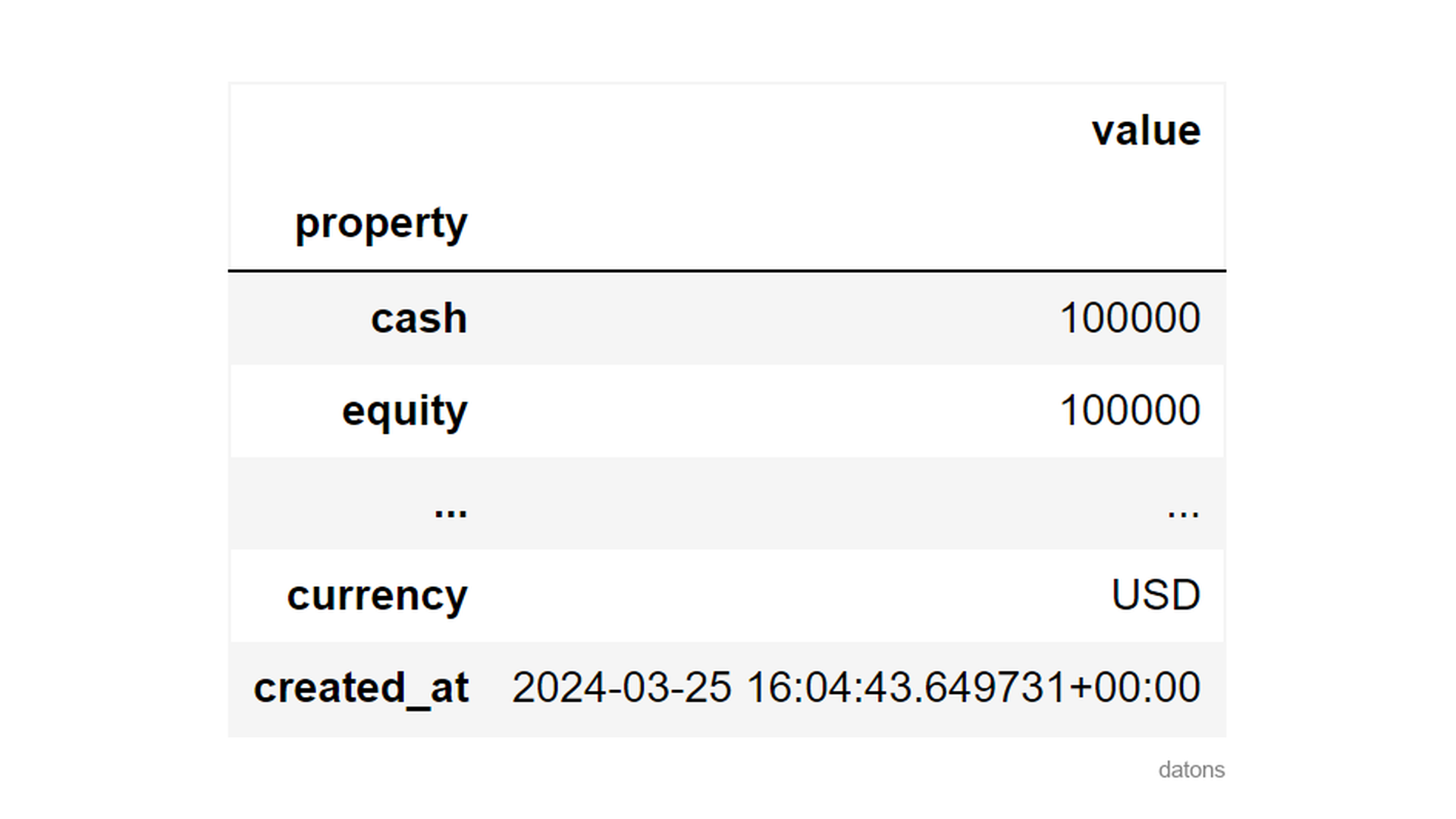
Request First Order
Let’s go all-in on Bitcoin BTCUSD
with the
available balance.
To register the order, create a MarketOrderRequest
object specifying the conditions.
notional
is the amount of money you want to invest in the order. If you want to buy a specific number of shares, you should use the qty parameter.GTC
Good Till Cancelled indicates that the order will remain active until executed.
order = requests.MarketOrderRequest(
symbol='BTCUSD',
notional=100_000,
side=enums.OrderSide.BUY,
time_in_force=enums.TimeInForce.GTC,
)
client.submit_order(order)
If you visit your active orders, you’ll see the buy order for Bitcoin.
Would this be interesting to one of your friends? Share it with them.
In our case, the order was executed by buying 1.40 Bitcoins at an average price of $69,837.9552.
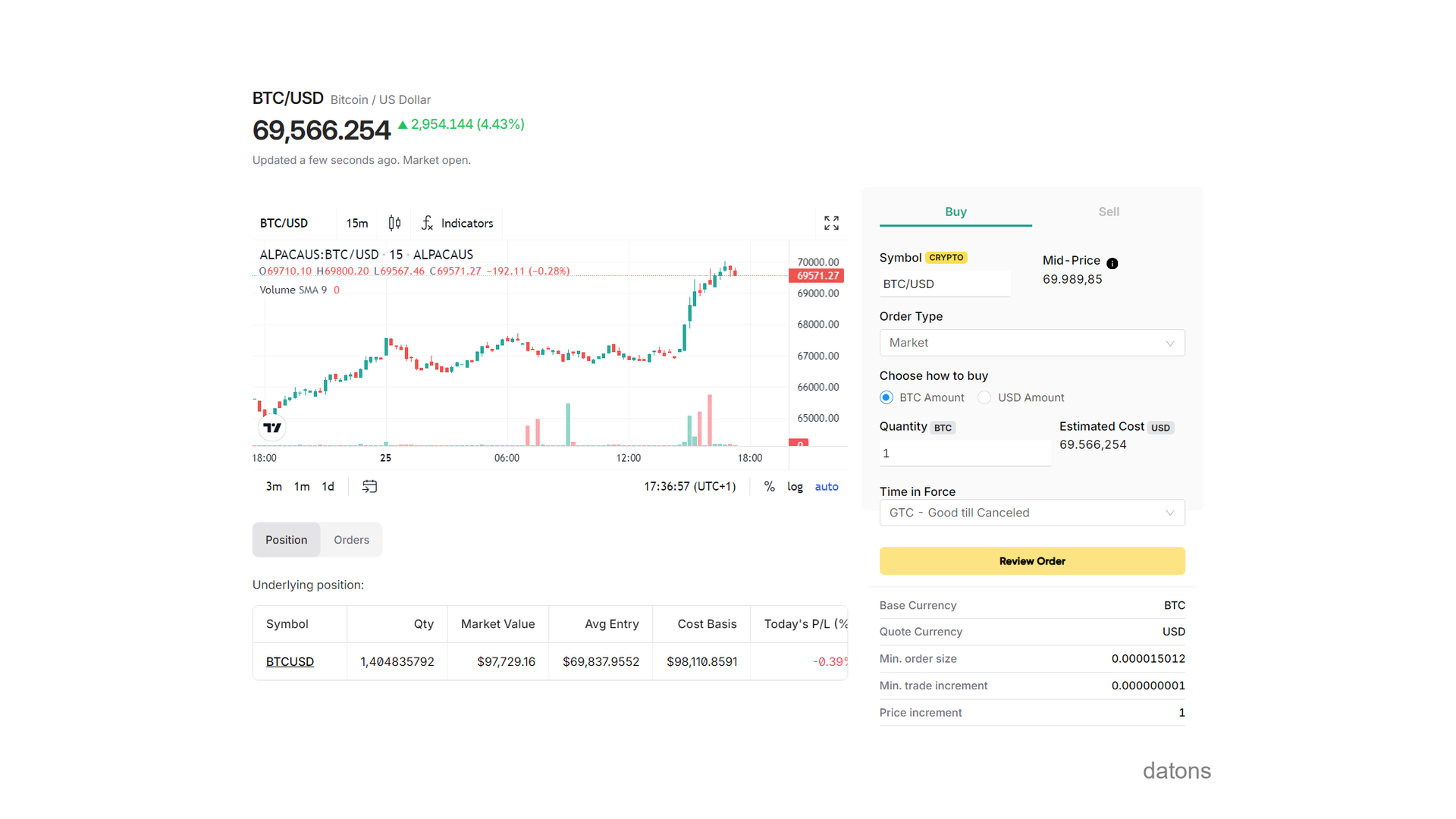
The execution of the order in Bitcoin should be immediate. However, for other financial assets, the order may take time to execute.
Close Asset Position
Now, you have an asset as an open position in your account.
To close the position, you’ll need the asset_id
of the
asset.
position = client.get_open_position('BTCUSD')
client.close_position(symbol_or_asset_id=position.asset_id)
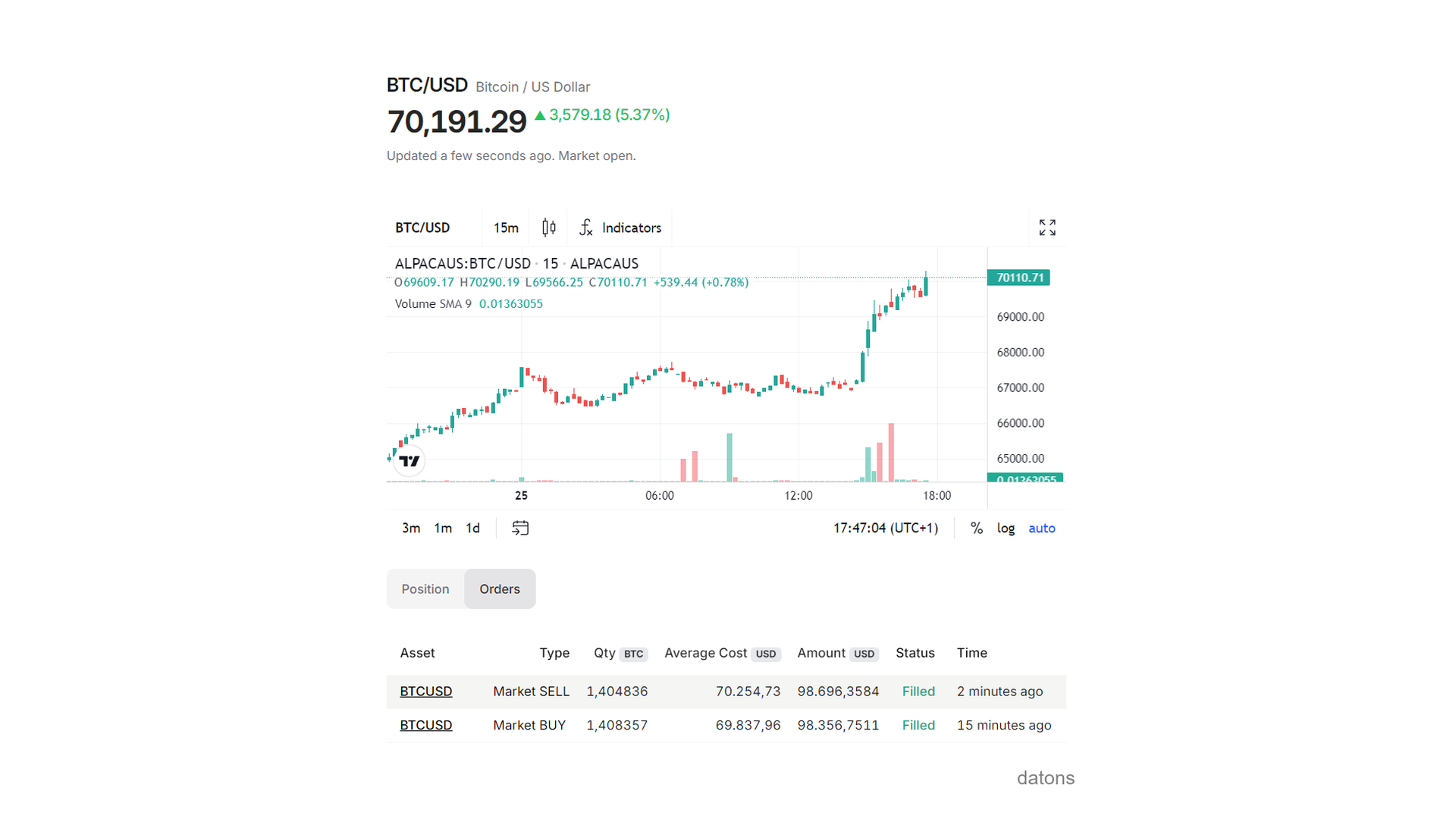
Portfolio Balance
Finally, you’ll observe your portfolio with the updated balance on the Alpaca platform.
We noticed that the balance increased by $92.86 after the operations.
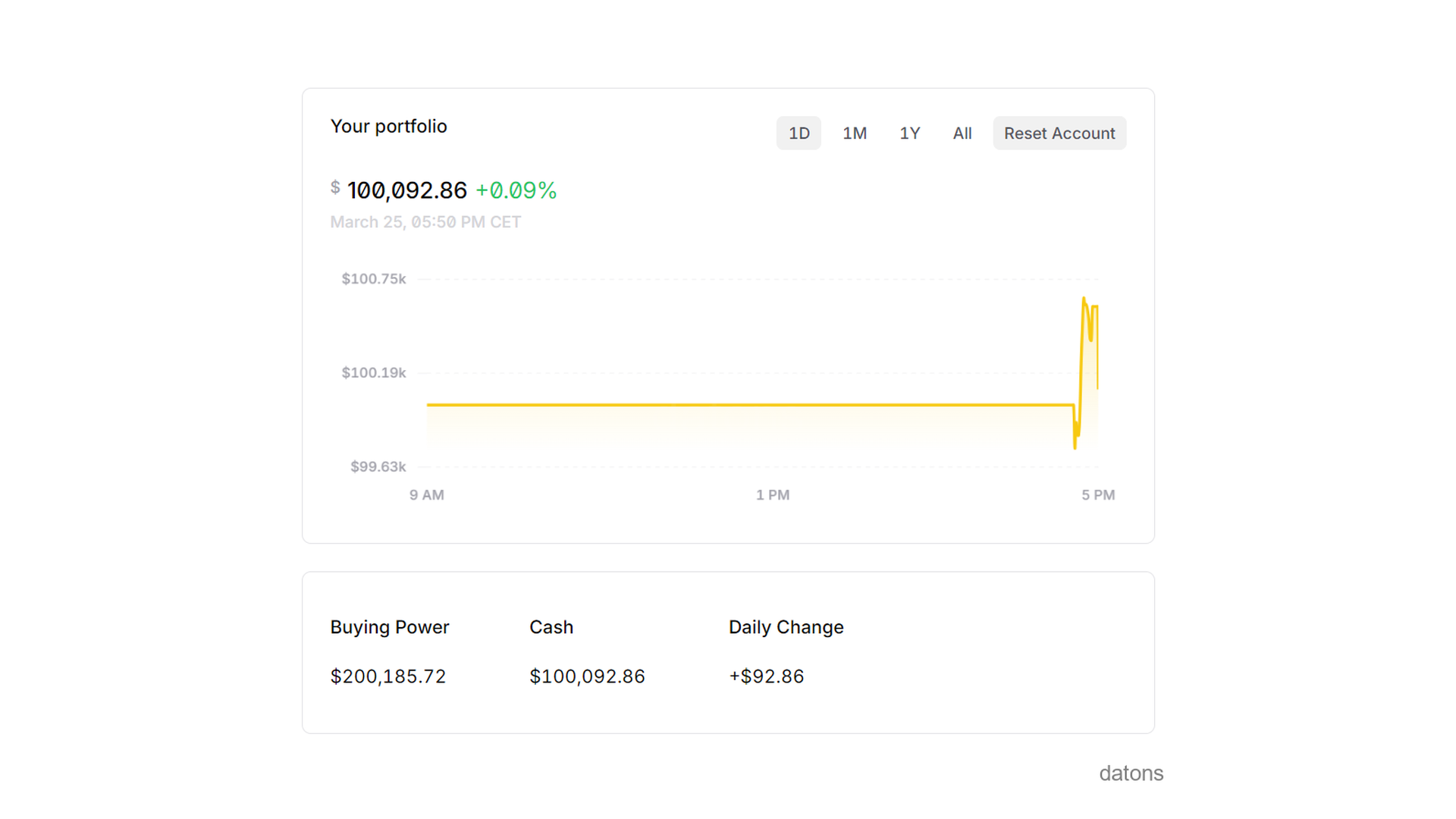
And that’s how you can use programming to make decisions that are reflected in reality using APIs.
Questions? Let’s talk below in the comments.
Conclusions
- Authorization for Trading Operations:
TradingClient
in demo modepaper=True
for operating with the trading API. - Demo Account Information:
client.get_account
retrieves information from the demo account, including balance and status. - Creation of a Buy Order:
client.submit_order
creates and sends aMarketOrderRequest
buy order for an asset specifying details such as amount and price. - Use of GTC in Orders:
enums.TimeInForce.GTC
establishes that the order remains active until executed. - Closing an Open Position:
client.close_position
closes an open position for a specific asset. - Updated Visualization of Operations: all information about the operations performed is reflected on the Alpaca platform.
If you could program whatever you wanted, what would it be? The upcoming tutorial might cover it ;)
Let’s talk in the comments below.